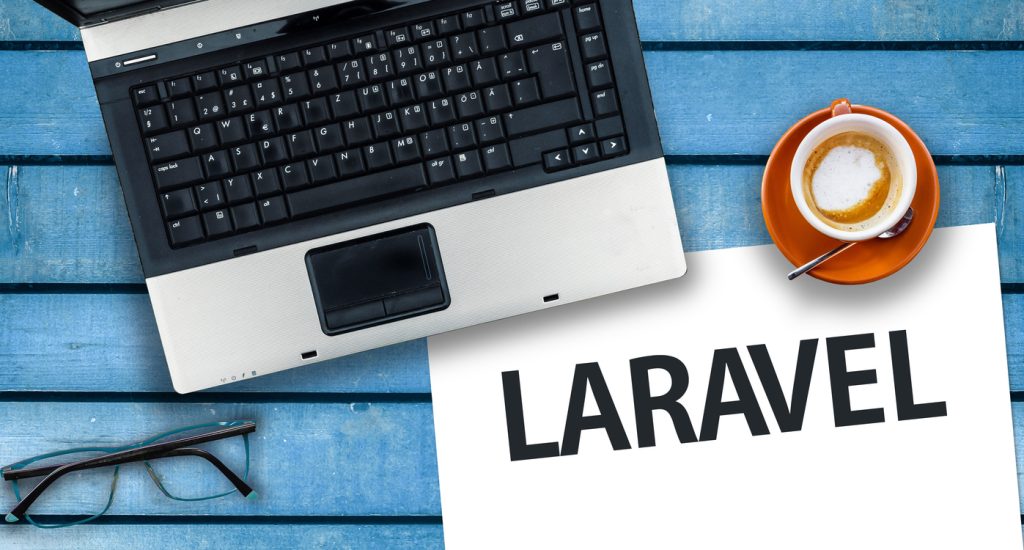
Progressive Web Applications (PWAs) are becoming increasingly popular due to their ability to offer a seamless and engaging user experience. Combining the best features of web and mobile applications, PWAs are fast, reliable, and installable, providing users with an app-like experience directly from their web browsers. Laravel, a robust PHP framework, makes building PWAs more straightforward and efficient. This guide will walk you through the steps to create a PWA with Laravel.
The Advantages of Developing a PWA with the Laravel Framework
Creating Progressive Web Applications (PWAs) using the Laravel framework offers numerous benefits, leveraging Laravel’s robust features:
Seamless Performance and Speed
- Efficient Routing and Caching: Laravel’s routing system and built-in caching mechanisms ensure fast loading times and efficient performance.
- Eloquent ORM: Laravel’s Eloquent ORM simplifies database interactions, speeding up data retrieval and manipulation.
Enhanced User Engagement
- Robust Authentication and Authorization: Laravel provides built-in tools for secure user authentication and authorization.
- Real-time Event Broadcasting: Laravel’s event broadcasting allows for real-time updates, enhancing user interaction.
Cost-Effective Development
- Rapid Development: Laravel’s elegant syntax and comprehensive documentation facilitate rapid development.
- Reusable Components: Laravel’s modular structure allows for reusable components, reducing development time and costs.
Enhanced Security
- Built-in Security Features: Laravel includes protections against SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- HTTPS by Default: Laravel encourages the use of HTTPS, ensuring secure data transmission.
Improved SEO and Discoverability
- SEO-friendly URLs: Laravel’s routing system supports SEO-friendly URLs, improving search engine rankings.
- Meta Tag Management: Laravel makes it easy to manage meta tags and other SEO elements.
Superior Developer Experience
- Comprehensive Documentation: Laravel offers extensive and well-organized documentation.
- Active Community: Laravel has a large, active community providing support, packages, and tools.
Prerequisites
Before we start, ensure you have the following installed on your system:
- PHP (>= 7.2)
- Composer
- Node.js and npm
- Laravel CLI
Step 1: Setting up a New Laravel Project
First, create a new Laravel project using Composer:
composer create-project --prefer-dist laravel/laravel pwa-laravel
Navigate to the project directory:
cd pwa-laravel
Step 2: Setting Up a Laravel PWA Package
To quickly scaffold the PWA features, we will use a Laravel PWA package. One popular package is laravel-pwa
.
Install the package using Composer:
composer require ladumor/laravel-pwa
After installation, publish the configuration file:
php artisan laravel-pwa:publish
This command will create a configuration file (config/pwa.php
) where you can customize your PWA settings.
Step 3: Configuring the PWA
Open the config/pwa.php
file and configure your PWA settings. Below is an example configuration:
return [
'name' => 'My Laravel PWA',
'short_name' => 'LaravelPWA',
'start_url' => '/',
'background_color' => '#ffffff',
'theme_color' => '#007bff',
'display' => 'standalone',
'orientation' => 'any',
'icons' => [
'72x72' => [
'path' => '/images/icons/icon-72x72.png',
'purpose' => 'any'
],
'96x96' => [
'path' => '/images/icons/icon-96x96.png',
'purpose' => 'any'
],
'128x128' => [
'path' => '/images/icons/icon-128x128.png',
'purpose' => 'any'
],
'144x144' => [
'path' => '/images/icons/icon-144x144.png',
'purpose' => 'any'
],
'152x152' => [
'path' => '/images/icons/icon-152x152.png',
'purpose' => 'any'
],
'192x192' => [
'path' => '/images/icons/icon-192x192.png',
'purpose' => 'any'
],
'384x384' => [
'path' => '/images/icons/icon-384x384.png',
'purpose' => 'any'
],
'512x512' => [
'path' => '/images/icons/icon-512x512.png',
'purpose' => 'any'
],
],
'offline' => [
'page' => 'offline'
]
];
Step 4: Adding Service Worker
Service workers are at the core of any PWA, enabling features like offline access and push notifications. The laravel-pwa
package automatically generates a service worker file for you.
To customize your service worker, you can edit the public/serviceworker.js
file. For a basic setup, you might not need to make significant changes here, but you can add custom caching strategies or background sync as needed.
Step 5: Creating the Manifest File
The manifest file is a JSON file that tells the browser about your PWA and how it should behave when installed on a device. The laravel-pwa
package automatically generates the manifest file based on your configuration in pwa.php
.
To customize the manifest, you can modify the config/pwa.php
file or directly edit the generated public/manifest.json
file.
Step 6: Handling Offline Pages
Create an offline page that will be shown when the user is offline. Create a new Blade template resources/views/offline.blade.php
and add your offline message:
<!DOCTYPE html>
<html>
<head>
<title>Offline</title>
</head>
<body>
<h1>You're offline</h1>
<p>Please check your internet connection and try again.</p>
</body>
</html>
In your web.php
routes file, add a route to handle the offline page:
Route::get('/offline', function () {
return view('offline');
});
Step 7: Testing Your PWA
To test your PWA, ensure your development server is running:
php artisan serve
Visit http://localhost:8000
in your browser. Open the browser’s developer tools and navigate to the “Application” tab. You should see your PWA manifest, service worker, and other settings configured correctly.
Step 8: Making Your PWA Installable
For a PWA to be installable, it needs to meet certain criteria, such as having a valid manifest file and serving all files over HTTPS. In a production environment, ensure your application is served over HTTPS. You can use services like Let’s Encrypt to obtain a free SSL certificate.
Step 9: Enhancing the PWA
Push Notifications
To add push notifications, you will need to integrate with a push service like Firebase Cloud Messaging (FCM). This involves configuring FCM, adding necessary scripts to your application, and handling push events in your service worker.
Background Sync
Background sync allows your PWA to defer actions until the user has a stable internet connection. This can be useful for sending data collected offline to the server once connectivity is restored. You can implement background sync by adding sync events to your service worker.
Conclusion
Building a Progressive Web Application with Laravel is a straightforward process, thanks to the robust tools and libraries available. By following this step-by-step guide, you can create a PWA that offers an enhanced user experience with offline capabilities, push notifications, and an app-like interface. As PWAs continue to grow in popularity, leveraging their features can provide significant benefits to your users and your business. Start building your Laravel PWA today and take advantage of the powerful combination of web and mobile technologies.